Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
Tags
- geth
- 스마트 컨트랙트
- 이더리움
- solidity
- NFT
- ERC20
- 블록체인
- web3.js
- MySQL
- blockchain
- ERC165
- tcp
- git
- 제어의역전
- truffle
- github
- ethers
- Docker
- erc
- Ethereum
- server
- Programming
- 트랜잭션
- web3
- 솔리디티
- 네트워크
- Python
- JavaScript
- web
- erc721
Archives
- Today
- Total
멍개의 연구소
[ethereum] mnemonic 생성, privatekey 복구와 계층적 구조의 원리 본문
· 라이브러리 설치
$ npm install --save bip39 $ npm install --save ethereumjs-wallet
· mnemonic 생성
const bip39 = require("bip39");
const mnemonic = bip39.generateMnemonic();
console.log(`mnemonic is : "${mnemonic}"`);
bip39를 이용하여 mneminic을 생성할 수 있습니다. mnemonic은 12개의 단어로 이루어진 문자열입니다.
· privatekey 복구
const bip39 = require("bip39");
const { hdkey } = require("ethereumjs-wallet");
const mnemonic = "good buyer welcome fabric call effort initial vendor surge diesel leader flip";
(async () => {
const seed = await bip39.mnemonicToSeed(mnemonic); // seed === entropy
const rootKey = hdkey.fromMasterSeed(seed);
const hardenedKey = rootKey.derivePath("m/44'/60'/0'/0");
const childKey = hardenedKey.deriveChild(0); // 값조정 가능
const wallet = childKey.getWallet();
const address = wallet.getAddress();
const privateKey = wallet.getPrivateKey();
console.log(`seed is ${seed.toString('hex')}`)
console.log(`======== rootKey =======`)
console.log(rootKey)
console.log(`======= childKey =======`)
console.log(childKey)
console.log(`======= wallet is =======`)
console.log(wallet)
console.log(`address is ${address.toString("hex")}`);
console.log(`privateKey is ${privateKey.toString("hex")}`);
})()
생성된 mnemonic을 이용하여 privatekey와 address를 생성할 수 있습니다.
mnemonic을 이용하면 항상 동일한 privatekey와 address를 생성할 수 있습니다. 정확히 말하면 childrenKey를생성하게 됩니다.
mnemonic을 이용하면 128Bits의 seed(entropy)를 생성합니다 seed를 이용하여 masterKey를 생성하며 마스터키를 이용하여 childrenKey 생성이 가능하며 해당 키를 이용하여 public, private key생성이 가능해집니다. 아래 이미지를 참고하면 좀 더 이해가 쉬울겁니다.
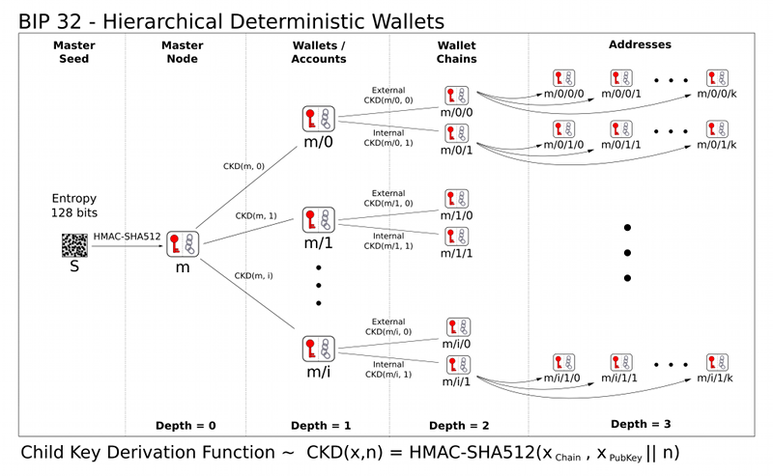
masterKey 또는 childrenKey의 derivePath를 이용하면 privateKey와 publicKey생성이 가능합니다.
이러한 구조를 계층구조라고 합니다
let hardenedKey1 = childKey.derivePath("m/44'/60'/0'/0");
let childKey1 = hardenedKey1.deriveChild(0)
console.log(childKey1.getWallet().getAddress().toString('hex'))
console.log(childKey1.getWallet().getPrivateKey().toString('hex'))
해당 코드처럼 앞에서 생성한 childrenKey를 이용하여 또다시 childrenKey 생성이 가능합니다.
여기서 한가지 의문점이 있을 수 있습니다.
바로 derivePath인데 해당 구문은 다음 이미지를 참조하면 이해할 수 있습니다.
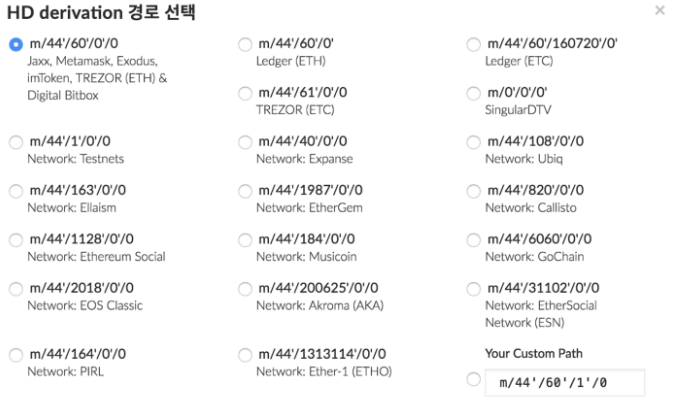
m / purpose' / coin_type' / account' / change / address_index
이더리움은 coin_type이 60입니다.
coin_type의 자세한 정보는 아래 링크에서 확인할 수 있습니다.
https://github.com/satoshilabs/slips/blob/master/slip-0044.md
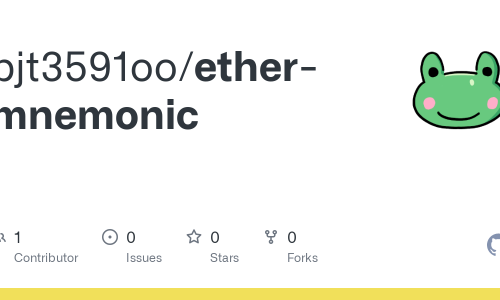
'블록체인' 카테고리의 다른 글
[ethereum] Geth를 이용하여 Clique(POA) 기반 private network 구축하기 (0) | 2022.08.28 |
---|---|
[ethereum] Geth를 이용하여 Ethash 기반 private network 구축하기 (0) | 2022.08.27 |
[ethereum] privatekey를 이용하여 address 복구, transaction 발생 (0) | 2022.08.27 |
[블록체인] 블록체인 시스템은 어떻게 구축할까 1편 - exchange, wallet, payment에서 transaction 취급방법 (0) | 2022.08.27 |
[블록체인] 거래소는 시세를 어떻게 형성할까? (0) | 2022.08.27 |
Comments