Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- ERC165
- solidity
- geth
- Programming
- github
- web3.js
- NFT
- ERC20
- 이더리움
- web3
- Ethereum
- 트랜잭션
- tcp
- JavaScript
- erc
- 솔리디티
- blockchain
- 제어의역전
- ethers
- Python
- server
- 네트워크
- MySQL
- erc721
- Docker
- truffle
- web
- git
- 스마트 컨트랙트
- 블록체인
Archives
- Today
- Total
멍개의 연구소
[ethereum] abi-decoder를 이용하여 inputData 해독하기 본문
안녕하세요. 멍개입니다.
abi-decoder 라이브러리를 이용하여 transaction에 포함된 inputData를 해석하는 방법을 다뤄보도록 하겠습니다.
· abi-decoder 모듈설치
$ npm install --save abi-decoder
npm을 이용하면 간단하게 설치할 수 있습니다.
· abi 추출하기
transaction의 inputData를 디코딩하기 위해서는 해당 컨트랙트의 ABI가 필요합니다.
pragma solidity 0.5;
contract test {
uint var1 = 10;
string var2 = "hello world";
function setData(uint a, uint b, string memory c) public {
var1 = a + b;
var2 = c;
}
function getData() public view returns(uint) {
return var1 + 10;
}
}
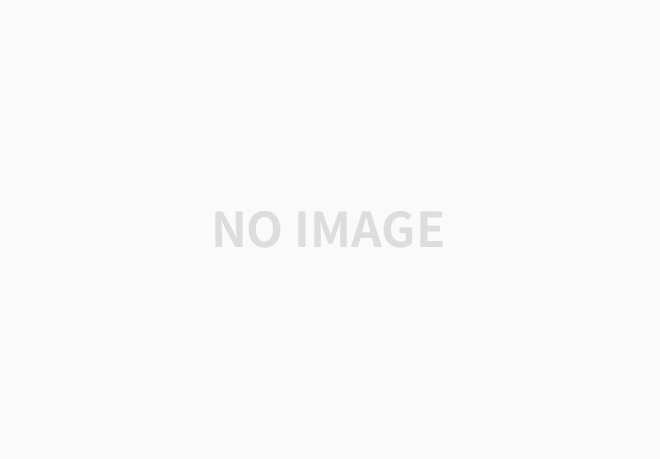
ABI 복사 버튼을 눌러줍니다.
[
{
"constant": true,
"inputs": [],
"name": "getData",
"outputs": [
{
"name": "",
"type": "uint256"
}
],
"payable": false,
"stateMutability": "view",
"type": "function"
},
{
"constant": false,
"inputs": [
{
"name": "a",
"type": "uint256"
},
{
"name": "b",
"type": "uint256"
},
{
"name": "c",
"type": "string"
}
],
"name": "setData",
"outputs": [],
"payable": false,
"stateMutability": "nonpayable",
"type": "function"
}
]
· transaction 발생하여 inputdata 얻기
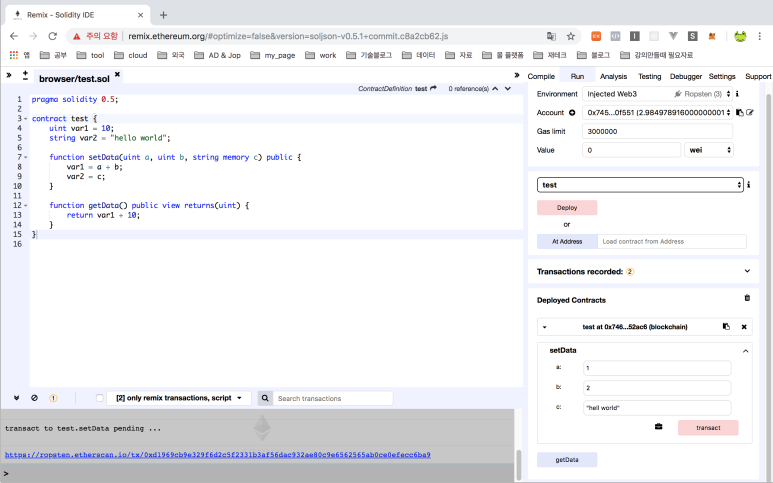
setData 함수를 호출하여 transaction을 발생합니다.
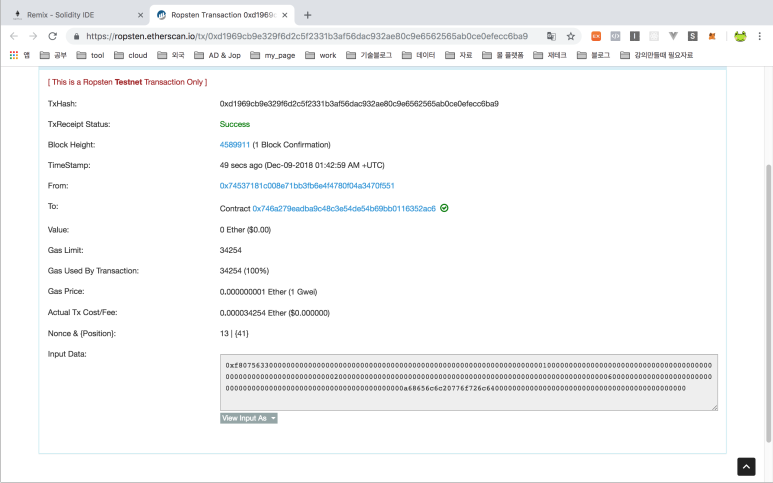
0xf8075633000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000020000000000000000000000000000000000000000000000000000000000000060000000000000000000000000000000000000000000000000000000000000000a68656c6c20776f726c6400000000000000000000000000000000000000000000
inputData는 to가 CA(contract address)라면 블록체인 네트워크에서 노드가 읽어서 EVM에서 실행하게 됩니다. 이제 inputData를 해독해보겠습니다.
· inputData 해독
앞에서 ABI와 transaction에 포함된 InputData를 복사합니다.
const abiDecoder = require('abi-decoder');
const testABI = [
{
"constant": true,
"inputs": [],
"name": "getData",
"outputs": [
{
"name": "",
"type": "uint256"
}
],
"payable": false,
"stateMutability": "view",
"type": "function"
},
{
"constant": false,
"inputs": [
{
"name": "a",
"type": "uint256"
},
{
"name": "b",
"type": "uint256"
},
{
"name": "c",
"type": "string"
}
],
"name": "setData",
"outputs": [],
"payable": false,
"stateMutability": "nonpayable",
"type": "function"
}
]
abiDecoder.addABI(testABI);
// testData엔 transaction의 inputData를 넣어줍니다.
const testData = "0xf80756330000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000200000000000000000000000000000000000000000000000000000000000000600000000000000000000000000000000000000000000000000000000000000006ed97aceba19c0000000000000000000000000000000000000000000000000000";
const decodedData = abiDecoder.decodeMethod(testData);
console.log(decodedData)
{ name: 'setData',
params:
[ { name: 'a', value: '1', type: 'uint256' },
{ name: 'b', value: '2', type: 'uint256' },
{ name: 'c', value: 'hell world', type: 'string' } ] }
실행결과를 보면 name엔 호출함수와 params엔 전달된 인자 정보를 볼 수 있습니다.
'블록체인' 카테고리의 다른 글
[블록체인] 거래소는 시세를 어떻게 형성할까? (0) | 2022.08.27 |
---|---|
[ethereum] transaction에 데이터를 포함하여 영원히 데이터 남기기 (1) | 2022.08.27 |
[ethereum] transaction을 보고 contract 생성을어떻게 할까 (0) | 2022.08.27 |
[ethereum] transaction 발생 시 - Warning! Error encountered during contract execution [Out of gas] 에러 (1) | 2022.08.27 |
[ethereum] solidity - address 관리방법(address, address payabl 타입) (0) | 2022.08.27 |